ASN1PEREncodeBuffer Class Reference
[PER Message Buffer Classes]
#include <asn1PerCppTypes.h>
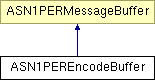
Public Member Functions | |
ASN1PEREncodeBuffer (OSBOOL aligned) | |
ASN1PEREncodeBuffer (OSOCTET *pMsgBuf, size_t msgBufLen, OSBOOL aligned) | |
ASN1PEREncodeBuffer (OSOCTET *pMsgBuf, size_t msgBufLen, OSBOOL aligned, OSRTContext *pContext) | |
EXTPERMETHOD | ASN1PEREncodeBuffer (OSRTOutputStream &ostream, OSBOOL aligned) |
int | byteAlign () |
int | encodeBit (OSBOOL value) |
int | encodeBits (const OSOCTET *pvalue, size_t nbits, OSUINT32 bitOffset=0) |
size_t | getMsgBitCnt () |
virtual EXTPERMETHOD OSOCTET * | getMsgCopy () |
virtual EXTPERMETHOD const OSOCTET * | getMsgPtr () |
EXTPERMETHOD int | init () |
virtual OSBOOL | isA (int bufferType) |
int | GetMsgBitCnt () |
Detailed Description
The ASN1PEREncodeBuffer class is derived from the ASN1PERMessageBuffer base class. It contains variables and methods specific to encoding ASN.1 messages. It is used to manage the buffer into which an ASN.1 PER message is to be encoded.
Constructor & Destructor Documentation
ASN1PEREncodeBuffer::ASN1PEREncodeBuffer | ( | OSBOOL | aligned | ) | [inline] |
This version of the ASN1PEREncodeBuffer constructor that takes one argument, aligned flag. It creates a dynamic memory buffer into which a PER message is encoded.
- Parameters:
-
aligned Flag indicating if aligned (TRUE) or unaligned (FALSE) encoding should be done.
ASN1PEREncodeBuffer::ASN1PEREncodeBuffer | ( | OSOCTET * | pMsgBuf, | |
size_t | msgBufLen, | |||
OSBOOL | aligned | |||
) | [inline] |
This version of the ASN1PEREncodeBuffer constructor takes a message buffer and size argument and an aligned flag argument (static encoding version).
- Parameters:
-
pMsgBuf A pointer to a fixed-size message buffer to receive the encoded message. msgBufLen Size of the fixed-size message buffer. aligned Flag indicating if aligned (TRUE) or unaligned (FALSE) encoding should be done.
ASN1PEREncodeBuffer::ASN1PEREncodeBuffer | ( | OSOCTET * | pMsgBuf, | |
size_t | msgBufLen, | |||
OSBOOL | aligned, | |||
OSRTContext * | pContext | |||
) | [inline] |
This version of the ASN1PEREncodeBuffer constructor takes a message buffer and size argument and an aligned flag argument (static encoding version) as well as a pointer to an existing context object.
- Parameters:
-
pMsgBuf A pointer to a fixed-size message buffer to receive the encoded message. msgBufLen Size of the fixed-size message buffer. aligned Flag indicating if aligned (TRUE) or unaligned (FALSE) encoding should be done. pContext A pointer to an OSRTContext structure created by the user.
EXTPERMETHOD ASN1PEREncodeBuffer::ASN1PEREncodeBuffer | ( | OSRTOutputStream & | ostream, | |
OSBOOL | aligned | |||
) |
This version of the ASN1PEREncodeBuffer constructor takes a reference to an output stream object and an aligned flag argument (stream encoding version).
- Parameters:
-
ostream A reference to an output stream object. aligned Flag indicating if aligned (TRUE) or unaligned (FALSE) encoding should be done.
Member Function Documentation
int ASN1PEREncodeBuffer::byteAlign | ( | ) | [inline] |
This method aligns the output buffer or stream to the next octet boundary.
- Returns:
- Status of operation: 0 = success, negative value if error occurred.
References pe_byte_align().
int ASN1PEREncodeBuffer::encodeBit | ( | OSBOOL | value | ) | [inline] |
This method writes a single encoded bit value to the output buffer or stream.
- Parameters:
-
value Boolean value of bit to be written.
- Returns:
- Status of operation: 0 = success, negative value if error occurred.
int ASN1PEREncodeBuffer::encodeBits | ( | const OSOCTET * | pvalue, | |
size_t | nbits, | |||
OSUINT32 | bitOffset = 0 | |||
) | [inline] |
This method writes the given number of bits from the byte array to the output buffer or stream starting from the given bit offset.
- Parameters:
-
pvalue Pointer to byte array containing data to be encoded. nbits Number of bits to copy from byte array to encode buffer. bitOffset Starting bit offset from which bits are to be copied.
- Returns:
- Status of operation: 0 = success, negative value if error occurred.
size_t ASN1PEREncodeBuffer::getMsgBitCnt | ( | ) | [inline] |
This method returns the length (in bits) of the encoded message.
- Returns:
- Length(in bits)of encoded message
References pe_GetMsgBitCnt().
virtual EXTPERMETHOD OSOCTET* ASN1PEREncodeBuffer::getMsgCopy | ( | ) | [virtual] |
This method returns a copy of the current encoded message. Memory is allocated for the message using the 'new' operation. It is the user's responsibility to free the memory using 'delete'.
- Returns:
- Pointer to copy of encoded message. It is the user's responsibility to release the memory using the 'delete' operator (i.e., delete [] ptr;)
virtual EXTPERMETHOD const OSOCTET* ASN1PEREncodeBuffer::getMsgPtr | ( | ) | [virtual] |
This method returns the internal pointer to the current encoded message.
- Returns:
- Pointer to encoded message.
EXTPERMETHOD int ASN1PEREncodeBuffer::init | ( | ) |
This method reinitializes the encode buffer pointer to allow a new message to be encoded. This makes it possible to reuse one message buffer object in a loop to encode multiple messages. After this method is called, any previously encoded message in the buffer will be overwritten on the next encode call.
- Returns:
- Completion status of operation:
- 0 (0) = success,
- negative return value is error.
virtual OSBOOL ASN1PEREncodeBuffer::isA | ( | int | bufferType | ) | [inline, virtual] |
This method checks the type of the message buffer.
- Parameters:
-
bufferType Enumerated identifier specifying a derived class. The only possible value for this class is PEREncode.
- Returns:
- Boolean result of the match operation. True if this is the class corresponding to the identifier argument.
The documentation for this class was generated from the following file: