ASN1BERDecodeBuffer Class Reference
[BER Message Buffer Classes]
#include <asn1BerCppTypes.h>
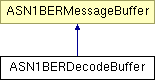
Public Member Functions | |
ASN1BERDecodeBuffer () | |
ASN1BERDecodeBuffer (const OSOCTET *pMsgBuf, size_t msgBufLen) | |
ASN1BERDecodeBuffer (const OSOCTET *pMsgBuf, size_t msgBufLen, OSRTContext *pContext) | |
OSOCTET * | findElement (ASN1TAG tag, OSINT32 &elemLen, OSBOOL firstFlag=TRUE) |
virtual const OSOCTET * | getMsgPtr () |
int | init () |
virtual OSBOOL | isA (Type bufferType) |
int | parseTagLen (ASN1TAG &tag, int &msglen) |
int | readBinaryFile (const char *filePath) |
int | setBuffer (const OSOCTET *pMsgBuf, size_t msgBufLen) |
OSOCTET * | FindElement (ASN1TAG tag, int &elemLen, int firstFlag=1) |
int | ParseTagLen (ASN1TAG &tag, int &msglen) |
ASN1BERDecodeBuffer & | operator>> (ASN1CType &val) |
Protected Attributes | |
const OSOCTET * | mpMsgBuf |
size_t | mMsgBufLen |
OSBOOL | mBufSetFlag |
Detailed Description
The ASN1BERDecodeBuffer class is derived from the ASN1BERMessageBuffer base class. It contains variables and methods specific to decoding ASN.1 BER/DER messages. It is used to manage the input buffer containing an ASN.1 message to be decoded.
Constructor & Destructor Documentation
ASN1BERDecodeBuffer::ASN1BERDecodeBuffer | ( | ) |
Default constructor. Use the getStatus() method to determine if an error occured during initialization or not.
ASN1BERDecodeBuffer::ASN1BERDecodeBuffer | ( | const OSOCTET * | pMsgBuf, | |
size_t | msgBufLen | |||
) |
Parameterized constructor. This constructor constructs a buffer describing an encoded ASN.1 message. Parameters describing the message to be decoded are passed as arguments.
- Parameters:
-
pMsgBuf A pointer to a buffer containing an encoded ASN.1 message. msgBufLen Size of the message buffer. This does not have to be equal to the length of the message. The message length can be determined from the outer tag-length-value in the message. This parameter is used to determine if the length of the message is valid; therefore it must be greater than or equal to the actual length. Typically, the size of the buffer the message was read into is passed.
ASN1BERDecodeBuffer::ASN1BERDecodeBuffer | ( | const OSOCTET * | pMsgBuf, | |
size_t | msgBufLen, | |||
OSRTContext * | pContext | |||
) |
Parameterized constructor. This constructor constructs a buffer describing an encoded ASN.1 message. Parameters describing the message to be decoded are passed as arguments.
- Parameters:
-
pMsgBuf A pointer to a buffer containing an encoded ASN.1 message. msgBufLen Size of the message buffer. This does not have to be equal to the length of the message. The message length can be determined from the outer tag-length-value in the message. This parameter is used to determine if the length of the message is valid; therefore it must be greater than or equal to the actual length. Typically, the size of the buffer the message was read into is passed. pContext A pointer to an existing OSRTContext structure.
Member Function Documentation
OSOCTET* ASN1BERDecodeBuffer::findElement | ( | ASN1TAG | tag, | |
OSINT32 & | elemLen, | |||
OSBOOL | firstFlag = TRUE | |||
) |
This method finds a tagged element within a message.
Calling Sequence:
ptr = decodeBuffer.findElement (tag, elemLen, firstFlag);
where decodeBuffer is an ASN1BERDecodeBuffer object.
- Returns:
- Pointer to tagged component in message or NULL if component not found.
- Parameters:
-
tag ASN.1 tag value to search for. firstFlag Flag indicating if this the first time this search is being done. If true, internal pointers will be set to start the search from the beginning of the message. If false, the search will be resumed from the point at which the last matching tag was found. This makes it possible to find all instances of a particular tagged element within a message elemLen Reference to an integer value to receive the length of the found element.
virtual const OSOCTET* ASN1BERDecodeBuffer::getMsgPtr | ( | ) | [inline, virtual] |
This method returns the internal pointer to the current message that has been set to be decoded.
- Returns:
- Pointer to message.
int ASN1BERDecodeBuffer::init | ( | ) |
Initializes message buffer.
- Returns:
- Completion status of operation:
- 0 (0) = success,
- negative return value is error.
virtual OSBOOL ASN1BERDecodeBuffer::isA | ( | Type | bufferType | ) | [inline, virtual] |
This method checks the type of the message buffer.
- Parameters:
-
bufferType Enumerated identifier specifying a derived class. The only possible value for this class is BERDecode.
- Returns:
- Boolean result of the match operation. True if this is the class corresponding to the identifier argument.
ASN1BERDecodeBuffer& ASN1BERDecodeBuffer::operator>> | ( | ASN1CType & | val | ) |
This operator decodes an instance of an ASN1CType derived class. Use the getStatus() method to determine if an error occured during the operation or not.
int ASN1BERDecodeBuffer::parseTagLen | ( | ASN1TAG & | tag, | |
int & | msglen | |||
) | [inline] |
This method will parse the initial tag-length pair from the message.
Calling Sequence:
stat = decodeBuffer.parseTagLen (tag, msglen);
where decodeBuffer is an ASN1BERDecodeBuffer object.
- Returns:
- Status of the operation. Possible values are 0 if successful or one of the negative error status codes defined in Appendix A of the C/C++ Common Functions Reference Manual.
- Parameters:
-
tag Reference to a tag structure to receive the outer level tag value parsed from the message. msglen Length of the message. This is the total length of the message obtained by adding the number of bytes in initial tag-length to the parsed length value.
References xd_setp().
int ASN1BERDecodeBuffer::readBinaryFile | ( | const char * | filePath | ) |
This method reads a file containing a single BER/DER/CER encoded data record into the buffer for decoding.
- Parameters:
-
filePath The zero-terminated string containing the path to the file.
- Returns:
- Completion status of operation:
- 0 (0) = success,
- negative return value is error.
int ASN1BERDecodeBuffer::setBuffer | ( | const OSOCTET * | pMsgBuf, | |
size_t | msgBufLen | |||
) |
This method sets a buffer containing a message to be decoded.
- Parameters:
-
pMsgBuf A pointer to a memory buffer containing a message to be decoded. The buffer should be declared as an array of unsigned characters (OSOCTETs). msgBufLen The length of the memory buffer in bytes.
- Returns:
- Completion status of operation:
- 0 (0) = success,
- negative return value is error.
The documentation for this class was generated from the following file: