ASN1BEREncodeBuffer Class Reference
[BER Message Buffer Classes]
#include <asn1BerCppTypes.h>
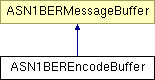
Public Member Functions | |
ASN1BEREncodeBuffer () | |
ASN1BEREncodeBuffer (OSOCTET *pMsgBuf, size_t msgBufLen) | |
ASN1BEREncodeBuffer (OSOCTET *pMsgBuf, size_t msgBufLen, OSRTContext *pContext) | |
void | freeBuffer () |
virtual OSOCTET * | getMsgCopy () |
virtual const OSOCTET * | getMsgPtr () |
int | init () |
virtual OSBOOL | isA (Type bufferType) |
int | setBuffer (OSOCTET *pMsgBuf, size_t msgBufLen) |
ASN1BEREncodeBuffer & | operator<< (ASN1CType &val) |
Detailed Description
The ANS1BEREncodeBuffer class is derived from the ASN1BERMessageBuffer base class. It contains variables and methods specific to encoding ASN.1 messages using the Basic Encoding Rules (BER). It is used to manage the buffer into which an ASN.1 message is to be encoded.
Constructor & Destructor Documentation
ASN1BEREncodeBuffer::ASN1BEREncodeBuffer | ( | ) |
Default constructor. This sets all internal variables to their initial values. Use the getStatus() method to determine if an error occured during initialization or not.
ASN1BEREncodeBuffer::ASN1BEREncodeBuffer | ( | OSOCTET * | pMsgBuf, | |
size_t | msgBufLen | |||
) |
Parameterized constructor. This version takes a message buffer and size argument (static encoding version). Use the getStatus() method to determine if an error occured during initialization or not.
- Parameters:
-
pMsgBuf A pointer to a fixed size message buffer to recieve the encoded message. msgBufLen Size of the fixed-size message buffer.
ASN1BEREncodeBuffer::ASN1BEREncodeBuffer | ( | OSOCTET * | pMsgBuf, | |
size_t | msgBufLen, | |||
OSRTContext * | pContext | |||
) |
Parameterized constructor. This version takes a message buffer, a size argument (static encoding version), and a context. Use the getStatus() method to determine if an error occured during initialization or not.
- Parameters:
-
pMsgBuf A pointer to a fixed size message buffer to recieve the encoded message. msgBufLen Size of the fixed-size message buffer. pContext A pointer to an existing OSRTContext structure.
Member Function Documentation
void ASN1BEREncodeBuffer::freeBuffer | ( | ) | [inline] |
This method releases memory that was allocated for a dynamic encode buffer.
References xe_free().
virtual OSOCTET* ASN1BEREncodeBuffer::getMsgCopy | ( | ) | [virtual] |
This method returns a copy of the current encoded message. Memory is allocated for the message using the 'new []' operation. It is the users's responsibility to free the memory using 'delete []'.
Calling Sequence:
ptr = encodeBuffer.getMsgCopy ();
where encodeBuffer is an ASN1BEREncodeBuffer object.
- Returns:
- Pointer to copy of encoded message. It is the users's responsibility to free the memory using 'delete []' (i.e., delete [] ptr;).
virtual const OSOCTET* ASN1BEREncodeBuffer::getMsgPtr | ( | ) | [virtual] |
This method returns the internal pointer to the current encoded message. Calling Sequence:
ptr = encodeBuffer.getMsgPtr ();
where encodeBuffer is an ASN1BEREncodeBuffer object.
- Returns:
- Pointer to encoded message.
int ASN1BEREncodeBuffer::init | ( | ) |
This method reinitializes the encode buffer pointer to allow a new message to be encoded. This makes it possible to reuse one message buffer object in a loop to encode multiple messages. After this method is called, any previously encoded message in the buffer will be overwritten on the next encode call.
Calling Sequence:
encodeBuffer.init ();
where encodeBuffer is an ASN1BEREncodeBuffer object.
- Returns:
- Completion status of operation:
- 0 (0) = success,
- negative return value is error.
virtual OSBOOL ASN1BEREncodeBuffer::isA | ( | Type | bufferType | ) | [inline, virtual] |
This method checks the type of the message buffer.
- Parameters:
-
bufferType Enumerated identifier specifying a derived class. The only possible value for this class is BEREncode.
- Returns:
- Boolean result of the match operation. True if this is the class corresponding to the identifier argument.
ASN1BEREncodeBuffer& ASN1BEREncodeBuffer::operator<< | ( | ASN1CType & | val | ) |
This operator encodes instance of ASN1CType derived class. Use getStatus() method to determine has error occured during the operation or not.
int ASN1BEREncodeBuffer::setBuffer | ( | OSOCTET * | pMsgBuf, | |
size_t | msgBufLen | |||
) |
This method sets a buffer to receive the encoded message.
- Parameters:
-
pMsgBuf A pointer to a memory buffer to use to encode a message. The buffer should be declared as an array of unsigned characters (OSOCTETs). This parameter can be set to NULL to specify dynamic encoding (i.e., the encode functions will dynamically allocate a buffer for the message). msgBufLen The length of the memory buffer in bytes. If pMsgBuf is NULL, this parameter specifies the initial size of the dynamic buffer; if 0 - the default size will be used.
- Returns:
- Completion status of operation:
- 0 (0) = success,
- negative return value is error.
The documentation for this class was generated from the following file: