ASN1CSeqOfList Class Reference
[Control (ASN1C_) Base Classes]
#include <ASN1CSeqOfList.h>
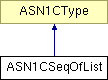
Public Member Functions | |
EXTRTMETHOD | ASN1CSeqOfList (OSRTMessageBufferIF &msgBuf, OSRTDList &lst, OSBOOL initBeforeUse=TRUE) |
EXTRTMETHOD | ASN1CSeqOfList (OSRTMessageBufferIF &msgBuf) |
EXTRTMETHOD | ASN1CSeqOfList (ASN1CType &ccobj) |
EXTRTMETHOD | ASN1CSeqOfList (OSRTMessageBufferIF &msgBuf, ASN1TSeqOfList &lst) |
EXTRTMETHOD | ASN1CSeqOfList (ASN1CType &ccobj, ASN1TSeqOfList &lst) |
EXTRTMETHOD | ASN1CSeqOfList (OSRTMessageBufferIF &msgBuf, ASN1TPDUSeqOfList &lst) |
EXTRTMETHOD | ASN1CSeqOfList (OSRTContext &ctxt, OSRTDList &lst, OSBOOL initBeforeUse=TRUE) |
EXTRTMETHOD | ASN1CSeqOfList (OSRTContext &ctxt) |
EXTRTMETHOD | ASN1CSeqOfList (OSRTContext &ctxt, ASN1TSeqOfList &lst) |
EXTRTMETHOD | ASN1CSeqOfList (OSRTContext &ctxt, ASN1TPDUSeqOfList &lst) |
EXTRTMETHOD void | append (void *data) |
EXTRTMETHOD void | appendArray (const void *data, OSSIZE numElems, OSSIZE elemSize) |
EXTRTMETHOD void | appendArrayCopy (const void *data, OSSIZE numElems, OSSIZE elemSize) |
void | init () |
EXTRTMETHOD void | insert (int index, void *data) |
EXTRTMETHOD void | remove (int index) |
EXTRTMETHOD void | remove (void *data) |
void | removeFirst () |
void | removeLast () |
EXTRTMETHOD int | indexOf (void *data) const |
OSBOOL | contains (void *data) const |
EXTRTMETHOD void * | getFirst () |
EXTRTMETHOD void * | getLast () |
EXTRTMETHOD void * | get (int index) const |
EXTRTMETHOD void * | set (int index, void *data) |
EXTRTMETHOD void | clear () |
EXTRTMETHOD void | freeMemory () |
EXTRTMETHOD OSBOOL | isEmpty () const |
EXTRTMETHOD OSSIZE | size () const |
EXTRTMETHOD ASN1CSeqOfListIterator * | iterator () |
EXTRTMETHOD ASN1CSeqOfListIterator * | iteratorFromLast () |
EXTRTMETHOD ASN1CSeqOfListIterator * | iteratorFrom (void *data) |
EXTRTMETHOD void * | toArray (OSSIZE elemSize) |
EXTRTMETHOD void * | toArray (void *pArray, OSSIZE elemSize, OSSIZE allocatedElems) |
void * | operator[] (int index) const |
operator OSRTDList * () | |
Protected Member Functions | |
EXTRTMETHOD | ASN1CSeqOfList (OSRTDList &lst) |
EXTRTMETHOD | ASN1CSeqOfList (ASN1TSeqOfList &lst) |
EXTRTMETHOD | ASN1CSeqOfList (ASN1TPDUSeqOfList &lst) |
EXTRTMETHOD void | remove (OSRTDListNode *node) |
EXTRTMETHOD void | insertBefore (void *data, OSRTDListNode *node) |
EXTRTMETHOD void | insertAfter (void *data, OSRTDListNode *node) |
Protected Attributes | |
OSRTDList * | pList |
volatile int | modCount |
OSBOOL | wasAssigned |
Detailed Description
Doubly-linked list implementation. This class provides all functionality necessary for linked list operations. It is the base class for ASN1C compiler-generated ASN1C_ control classes for SEQUENCE OF and SET OF PDU types.
Constructor & Destructor Documentation
EXTRTMETHOD ASN1CSeqOfList::ASN1CSeqOfList | ( | OSRTMessageBufferIF & | msgBuf, | |
OSRTDList & | lst, | |||
OSBOOL | initBeforeUse = TRUE | |||
) |
This constructor creates a linked list using the OSRTDList argument. The constructor does not deep-copy the variable; it assigns a reference to it to an external variable.
The object will then directly operate on the given list variable.
- Parameters:
-
msgBuf Reference to an ASN1Message buffer derived object (for example, an ASN1BEREncodeBuffer). lst Reference to a linked list structure. initBeforeUse Set to TRUE if the passed linked list needs to be initialized (rtxDListInit to be called).
EXTRTMETHOD ASN1CSeqOfList::ASN1CSeqOfList | ( | OSRTMessageBufferIF & | msgBuf | ) |
This constructor creates an empty linked list.
- Parameters:
-
msgBuf Reference to an ASN1Message buffer derived object (for example, an ASN1BEREncodeBuffer).
EXTRTMETHOD ASN1CSeqOfList::ASN1CSeqOfList | ( | ASN1CType & | ccobj | ) |
This constructor creates an empty linked list.
- Parameters:
-
ccobj Reference to a control class object (for example, any generated ASN1C_ class object).
EXTRTMETHOD ASN1CSeqOfList::ASN1CSeqOfList | ( | OSRTMessageBufferIF & | msgBuf, | |
ASN1TSeqOfList & | lst | |||
) |
This constructor creates a linked list using the ASN1TSeqOfList (holder of OSRTDList) argument.
The construction does not deep-copy the variable, it assigns a reference to it to an internal variable. The object will then directly operate on the given list variable. This constructor is used with a compiler-generated linked list variable.
- Parameters:
-
msgBuf Reference to an ASN1Message buffer derived object (for example, an ASN1BEREncodeBuffer). lst Reference to a linked list holder.
EXTRTMETHOD ASN1CSeqOfList::ASN1CSeqOfList | ( | ASN1CType & | ccobj, | |
ASN1TSeqOfList & | lst | |||
) |
This constructor creates a linked list using the ASN1TSeqOfList (holder of OSRTDList) argument.
The construction does not deep-copy the variable, it assigns a reference to it to an internal variable. The object will then directly operate on the given list variable. This constructor is used with a compiler-generated linked list variable.
- Parameters:
-
ccobj Reference to a control class object (for example, any generated ASN1C_ class object). lst Reference to a linked list holder.
EXTRTMETHOD ASN1CSeqOfList::ASN1CSeqOfList | ( | OSRTMessageBufferIF & | msgBuf, | |
ASN1TPDUSeqOfList & | lst | |||
) |
This constructor creates a linked list using the ASN1TPDUSeqOfList argument.
The construction does not deep-copy the variable, it assigns a reference to it to an internal variable. The object will then directly operate on the given list variable. This constructor is used with a compiler-generated linked list variable.
- Parameters:
-
msgBuf Reference to an ASN1Message buffer derived object (for example, an ASN1BEREncodeBuffer). lst Reference to a linked list holder.
Member Function Documentation
EXTRTMETHOD void ASN1CSeqOfList::append | ( | void * | data | ) |
This method appends an item to the linked list.
This item is represented by a void pointer that can point to an object of any type. The rtxMemAlloc function is used to allocate memory for the list node structure, therefore, all internal list memory will be released whenever rtxMemFree is called.
- Parameters:
-
data Pointer to a data item to be appended to the list.
- Returns:
- - none
EXTRTMETHOD void ASN1CSeqOfList::appendArray | ( | const void * | data, | |
OSSIZE | numElems, | |||
OSSIZE | elemSize | |||
) |
This method appends array items' pointers to a doubly linked list.
The rtxMemAlloc function is used to allocate memory for the list node structure, therefore all internal list memory will be released whenever the rtxMemFree is called. The data is not copied; it is just assigned to the node.
- Parameters:
-
data Pointer to source array to be appended to the list. numElems The number of elements in the source array. elemSize The size of one element in the array. Use the sizeof() operator to pass this parameter.
- Returns:
- - none
EXTRTMETHOD void ASN1CSeqOfList::appendArrayCopy | ( | const void * | data, | |
OSSIZE | numElems, | |||
OSSIZE | elemSize | |||
) |
This method appends array items into a doubly linked list.
The rtxMemAlloc function is used to allocate memory for the list node structure; therefore all internal list memory will be released whenever rtxMemFree is called. The data will be copied; the memory will be allocated using rtxMemAlloc.
- Parameters:
-
data Pointer to source array to be appended to the list. numElems The number of elements in the source array. elemSize The size of one element in the array. Use the sizeof() operator to pass this parameter.
- Returns:
- - none
EXTRTMETHOD void ASN1CSeqOfList::clear | ( | ) |
This method removes all items from the list.
OSBOOL ASN1CSeqOfList::contains | ( | void * | data | ) | const [inline] |
This method returns TRUE if this list contains the specified pointer. Note that a match is not done on the contents of each data item (i.e. what is pointed at by the pointer), only the pointer values.
- Parameters:
-
data - Pointer to data item.
- Returns:
- TRUE if this pointer value found in the list.
EXTRTMETHOD void ASN1CSeqOfList::freeMemory | ( | ) |
This method removes all items from the list and frees the associated memory.
EXTRTMETHOD void* ASN1CSeqOfList::get | ( | int | index | ) | const |
This method returns the item at the specified position in the list.
- Parameters:
-
index Index of the item to be returned.
- Returns:
- The item at the specified index in the list.
EXTRTMETHOD void* ASN1CSeqOfList::getFirst | ( | ) |
This method returns the first item from the list or null if there are no elements in the list.
- Returns:
- The first item of the list.
EXTRTMETHOD void* ASN1CSeqOfList::getLast | ( | ) |
This method returns the last item from the list or null if there are no elements in the list.
- Returns:
- The last item in the list.
EXTRTMETHOD int ASN1CSeqOfList::indexOf | ( | void * | data | ) | const |
This method returns the index in this list of the first occurrence of the specified item, or -1 if the list does not contain the time.
- Parameters:
-
data - Pointer to data item to searched.
- Returns:
- The index in this list of the first occurrence of the specified item, or -1 if the list does not contain the item.
void ASN1CSeqOfList::init | ( | ) | [inline] |
This method initializes the linked list structure.
References rtxDListInit().
EXTRTMETHOD void ASN1CSeqOfList::insert | ( | int | index, | |
void * | data | |||
) |
This method inserts an item into the linked list structure.
The item is represented by a void pointer that can point to an object of any type. The rtxMemAlloc function is used to allocate memory for the list node structure. All internal list memory will be released when the rtxMemFree function is called.
- Parameters:
-
index Index at which the specified item is to be inserted. data Pointer to data item to be appended to the list.
- Returns:
- - none
EXTRTMETHOD OSBOOL ASN1CSeqOfList::isEmpty | ( | ) | const |
This method returns TRUE if the list is empty.
- Returns:
- TRUE if this list is empty.
EXTRTMETHOD ASN1CSeqOfListIterator* ASN1CSeqOfList::iterator | ( | ) |
This method returns an iterator over the elements in the linked list in the sequence from the fist to the last.
- Returns:
- The iterator over this linked list.
EXTRTMETHOD ASN1CSeqOfListIterator* ASN1CSeqOfList::iteratorFrom | ( | void * | data | ) |
This method runs an iterator over the elements in this linked list starting from the specified item in the list.
- Parameters:
-
data The item of the list to be iterated first.
- Returns:
- The iterator over this linked list.
EXTRTMETHOD ASN1CSeqOfListIterator* ASN1CSeqOfList::iteratorFromLast | ( | ) |
This method creates a reverse iterator over the elements in this linked list in the sequence from last to first.
- Parameters:
-
- none
- Returns:
- The reverse iterator over this linked list.
void* ASN1CSeqOfList::operator[] | ( | int | index | ) | const [inline] |
This method is the overloaded operator[].
It returns the item at the specified position in the list.
- See also:
- get (int index)
EXTRTMETHOD void ASN1CSeqOfList::remove | ( | void * | data | ) |
This method removes the first occurrence of the node with specified data from the linked list structure.
The rtxMemAlloc function was used to allocate the memory for the list node structure, therefore, all internal list memory will be released whenever the rtxMemFree function is called.
- Parameters:
-
data - Pointer to the data item to be appended to the list.
EXTRTMETHOD void ASN1CSeqOfList::remove | ( | int | index | ) |
This method removed a node at the specified index from the linked list structure.
The rtxMemAlloc function was used to allocate the memory for the list node structure, therefore, all internal list memory will be released whenever the rtxMemFree is called.
- Parameters:
-
index Index of the item to be removed.
- Returns:
- - none
void ASN1CSeqOfList::removeFirst | ( | ) | [inline] |
This method removes the first node (head) from the linked list structure.
- Parameters:
-
- none
- Returns:
- - none
void ASN1CSeqOfList::removeLast | ( | ) | [inline] |
This method removes the last node (tail) from the linked list structure.
- Parameters:
-
- none
- Returns:
- - none
EXTRTMETHOD void* ASN1CSeqOfList::set | ( | int | index, | |
void * | data | |||
) |
This method replaces the item at the specified index in this list with the specified item.
- Parameters:
-
index The index of the item to be replaced. data The item to be stored at the specified index.
- Returns:
- The item previously at the specified position.
EXTRTMETHOD OSSIZE ASN1CSeqOfList::size | ( | ) | const |
This method returns the number of nodes in the list.
- Returns:
- The number of items in this list.
EXTRTMETHOD void* ASN1CSeqOfList::toArray | ( | void * | pArray, | |
OSSIZE | elemSize, | |||
OSSIZE | allocatedElems | |||
) |
This method converts the linked list into an array.
The rtxMemAlloc function is used to allocate memory for the array if the capacity of the specified array is exceeded.
- Parameters:
-
pArray Pointer to destination array. elemSize The size of one element in the array. Use the sizeof() operator to pass this parameter. allocatedElems The number of elements already allocated in the array. If this number is less than the number of nodes in the list, then a new array is allocated and returned. Memory is allocated using rtxMemAlloc function.
- Returns:
- The pointer to the converted array.
EXTRTMETHOD void* ASN1CSeqOfList::toArray | ( | OSSIZE | elemSize | ) |
This method converts the linked list into a new array.
The rtxMemAlloc function is used to allocate memory for an array.
- Parameters:
-
elemSize The size of one element in the array. Use the sizeof() operator to pass this parameter.
- Returns:
- The point to converted array.
The documentation for this class was generated from the following file: