ASN1XEREncodeStream Class Reference
[C++ classes for streaming XER encoding.]
#include <ASN1XEREncodeStream.h>
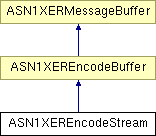
Public Member Functions | |
ASN1XEREncodeStream (OSRTOutputStreamIF &os, OSBOOL canonical=FALSE) | |
ASN1XEREncodeStream (OSRTOutputStreamIF *pos, OSBOOL bOwnStream=TRUE, OSBOOL canonical=FALSE) | |
virtual void * | getAppInfo () |
virtual OSRTCtxtPtr | getContext () |
virtual OSCTXT * | getCtxtPtr () |
virtual char * | getErrorInfo () |
virtual char * | getErrorInfo (char *pBuf, size_t &bufSize) |
virtual int | getStatus () const |
virtual void | printErrorInfo () |
virtual void | resetErrorInfo () |
virtual void | setAppInfo (void *pAppInfo) |
virtual void | setDiag (OSBOOL value=TRUE) |
virtual int | close () |
virtual int | flush () |
virtual OSBOOL | isOpened () |
virtual long | write (const OSOCTET *pdata, size_t size) |
ASN1XEREncodeStream & | operator<< (ASN1CType &val) |
int | encodeObj (ASN1CType &val) |
OSBOOL | isA (Type bufferType) |
Protected Attributes | |
OSRTOutputStreamIF * | mpStream |
OSBOOL | mbOwnStream |
Detailed Description
This class is a base class for other ASN.1 XER output stream's classes. It is derived from the ASN1Stream base class. It contains variables and methods specific to streaming encoding of XER messages.
Constructor & Destructor Documentation
ASN1XEREncodeStream::ASN1XEREncodeStream | ( | OSRTOutputStreamIF & | os, | |
OSBOOL | canonical = FALSE | |||
) |
A default constructor. Use getStatus() method to determine has error occured during the initialization or not.
Member Function Documentation
virtual int ASN1XEREncodeStream::close | ( | ) | [inline, virtual] |
Closes the input or output stream and releases any system resources associated with the stream. For output streams this function also flushes all internal buffers to the stream.
- Returns:
- Completion status of operation:
- 0 = success,
- negative return value is error.
- See also:
- rtxStreamClose
int ASN1XEREncodeStream::encodeObj | ( | ASN1CType & | val | ) |
This method encodes an ASN.1 constructed object to the stream.
- Parameters:
-
val A reference to an object to be encoded.
- Returns:
- Completion status of operation:
- 0 (0) = success,
- negative return value is error.
virtual int ASN1XEREncodeStream::flush | ( | ) | [inline, virtual] |
Flushes the buffered data to the stream.
- Returns:
- Completion status of operation:
- 0 = success,
- negative return value is error.
- See also:
- rtxStreamFlush
virtual void* ASN1XEREncodeStream::getAppInfo | ( | ) | [inline, virtual] |
Returns a pointer to application-specific information block
virtual OSRTCtxtPtr ASN1XEREncodeStream::getContext | ( | ) | [inline, virtual] |
The getContext method returns the underlying context smart-pointer object.
- Returns:
- Context smart pointer object.
virtual OSCTXT* ASN1XEREncodeStream::getCtxtPtr | ( | ) | [inline, virtual] |
The getCtxtPtr method returns the underlying C runtime context. This context can be used in calls to C runtime functions.
- Returns:
- The pointer to C runtime context.
virtual char* ASN1XEREncodeStream::getErrorInfo | ( | char * | pBuf, | |
size_t & | bufSize | |||
) | [inline, virtual] |
Returns error text in a memory buffer. If buffer pointer is specified in parameters (not NULL) then error text will be copied in the passed buffer. Otherwise, this method allocates memory using the 'operator new []' function. The calling routine is responsible to free the memory by using 'operator delete []'.
- Parameters:
-
pBuf A pointer to a destination buffer to obtain the error text. If NULL, dynamic buffer will be allocated. bufSize A reference to buffer size. If pBuf is NULL it will receive the size of allocated dynamic buffer.
- Returns:
- A pointer to a buffer with error text. If pBuf is not NULL, the return pointer will be equal to it. Otherwise, returns newly allocated buffer with error text. NULL, if error occured.
virtual char* ASN1XEREncodeStream::getErrorInfo | ( | ) | [inline, virtual] |
Returns error text in a dynamic memory buffer. Buffer will be allocated by 'operator new []'. The calling routine is responsible to free the memory by using 'operator delete []'.
- Returns:
- A pointer to a newly allocated buffer with error text.
virtual int ASN1XEREncodeStream::getStatus | ( | ) | const [inline, virtual] |
This method returns the completion status of previous operation. It can be used to check completion status of constructors or methods, which do not return completion status. If error occurs, use printErrorInfo method to print out the error's description and stack trace. Method resetError can be used to reset error to continue operations after recovering from the error.
- Returns:
- Runtime status code:
- 0 (0) = success,
- negative return value is error.
OSBOOL ASN1XEREncodeStream::isA | ( | Type | bufferType | ) | [virtual] |
This method matches an enumerated identifier defined in the base class. One identifier is declared for each of the derived classes.
- Parameters:
-
bufferType Enumerated identifier specifying a derived class. This type is defined as a public access type in the ASN1MessageBufferIF base interface. Possible values are: BEREncode, BERDecode, PEREncode, PERDecode, XEREncode, XERDecode, XMLEncode, XMLDecode, Stream.
- Returns:
- Boolean result of the match operation. True if this is the class corresponding to the identifier argument.
Reimplemented from ASN1XEREncodeBuffer.
virtual OSBOOL ASN1XEREncodeStream::isOpened | ( | ) | [inline, virtual] |
Checks, is the stream opened or not.
- Returns:
- TRUE, if the stream is opened, FALSE otherwise.
- See also:
- rtxStreamIsOpened
ASN1XEREncodeStream& ASN1XEREncodeStream::operator<< | ( | ASN1CType & | val | ) |
Encodes an ASN.1 constructed object to the stream. Use getStatus() method to determine has error occured during the operation or not.
- Parameters:
-
val A reference to an object to be encoded.
- Returns:
- reference to this class to perform sequential encoding.
virtual void ASN1XEREncodeStream::printErrorInfo | ( | ) | [inline, virtual] |
The printErrorInfo method prints information on errors contained within the context.
virtual void ASN1XEREncodeStream::resetErrorInfo | ( | ) | [inline, virtual] |
The resetErrorInfo method resets information on errors contained within the context.
virtual void ASN1XEREncodeStream::setAppInfo | ( | void * | pAppInfo | ) | [inline, virtual] |
Sets the application-specific information block.
virtual void ASN1XEREncodeStream::setDiag | ( | OSBOOL | value = TRUE |
) | [inline, virtual] |
The setDiag method will turn diagnostic tracing on or off.
- Parameters:
-
value - Boolean value (default = TRUE = on)
virtual long ASN1XEREncodeStream::write | ( | const OSOCTET * | pdata, | |
size_t | size | |||
) | [inline, virtual] |
Write data to the stream. This method writes the given number of octets from the given array to the output stream.
- Parameters:
-
pdata Pointer to the data to be written. size The number of octets to write.
- Returns:
- The total number of octets written into the stream, or negative value with error code if any error is occurred.
- See also:
- rtxStreamWrite
The documentation for this class was generated from the following file: