Asn1OerDecodeBuffer Class Reference
This class handles the decoding of ASN.1 messages as specified in the Octet Encoding Rules (OER) as documented in the ITU-T X.696 standard.
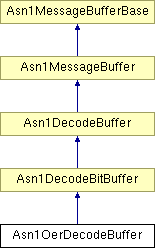
Public Member Functions | |
Asn1OerDecodeBuffer (System.IO.Stream istream) | |
Asn1OerDecodeBuffer (byte[] msgdata) | |
long | DecodeIntSigned () |
long | DecodeIntSigned (int octets) |
long | DecodeIntUnsigned () |
long | DecodeIntUnsigned (int octets) |
int | DecodeQuantity () |
void | DecodeTag (Asn1Tag tag) |
long | DecodeVarUnsigned (int octets) |
bool | GetCanonicalMode () |
void | SetCanonicalMode (bool value) |
Constructor & Destructor Documentation
Asn1OerDecodeBuffer | ( | byte[] | msgdata | ) |
This constructor creates a BER decode buffer object that references an encoded ASN.1 message.
- Parameters:
-
msgdata Byte array containing an encoded ASN.1 message.
Asn1OerDecodeBuffer | ( | System.IO.Stream | istream | ) |
This constructor creates a BER decode buffer object that references an encoded ASN.1 message.
In this case, the message is passed in using an InputStream object.
- Parameters:
-
istream Input stream containing an encoded ASN.1 message.
Member Function Documentation
long DecodeIntSigned | ( | ) |
Decode an integer value as a variable length, signed integer, including decoding the length, according to OER.
This is used for integer values that have a lower bound less than -2^63, no lower bound, or a lower bound less than zero in combination with an upper bound greater than 2^63–1, or no upper bound. (In other words, it doesn't fit in a signed 64-bit integer.)
long DecodeIntSigned | ( | int | octets | ) |
Decode a signed integer value (2's complement form), of the given length.
Note: this function will do sign-extension so that if a negative value was encoded in less than 8 bytes, the returned long will be that value (and not some positive value).
- Parameters:
-
octets; 0 < octets <= 8
- Returns:
long DecodeIntUnsigned | ( | ) |
Decode an integer value as a variable length, unsigned integer, including decoding the length, according to OER. This is used for integer values that are constrained to be non-negative but which have no upper bound or an upper bound greater than 2^64 - 1.
long DecodeIntUnsigned | ( | int | octets | ) |
Decode an unsigned integer value (a binary integer, not 2's complement form), of the given length. /p>
- Parameters:
-
octets The number of octets; 0 < octets <= 8
int DecodeQuantity | ( | ) |
Decode an OER quantity (used for SEQUENCE-OF and SET-OF).
- Returns:
- Exceptions:
-
IOException
void DecodeTag | ( | Asn1Tag | tag | ) |
Decode a tag encoded in OER.
- Parameters:
-
tag Object to decode into.
long DecodeVarUnsigned | ( | int | octets | ) |
Decode an unsigned integer value (a binary integer, not 2's complement form), of the given length.
This will throw an exception if canonical mode is set and the encoding used extra bytes.
Note: if octets >= 8, this method will throw an exception if the encoded integer is outside the range of a long; the exception is not necessarily thrown as the integer might not have been encoded in the minimal number of octets.
- Parameters:
-
octets The number of octets; 0 < octets
bool GetCanonicalMode | ( | ) |
Return true if canonical mode has been indicated by calling SetCanonicalMode(true);
void SetCanonicalMode | ( | bool | value | ) |
Turn canonical mode on/off. Turning canonical mode on acts as a signal to both generated code and runtime code that the user wants to enforce the canonical OER rules. It is not required to turn on canonical mode just because the encoding is canonical.